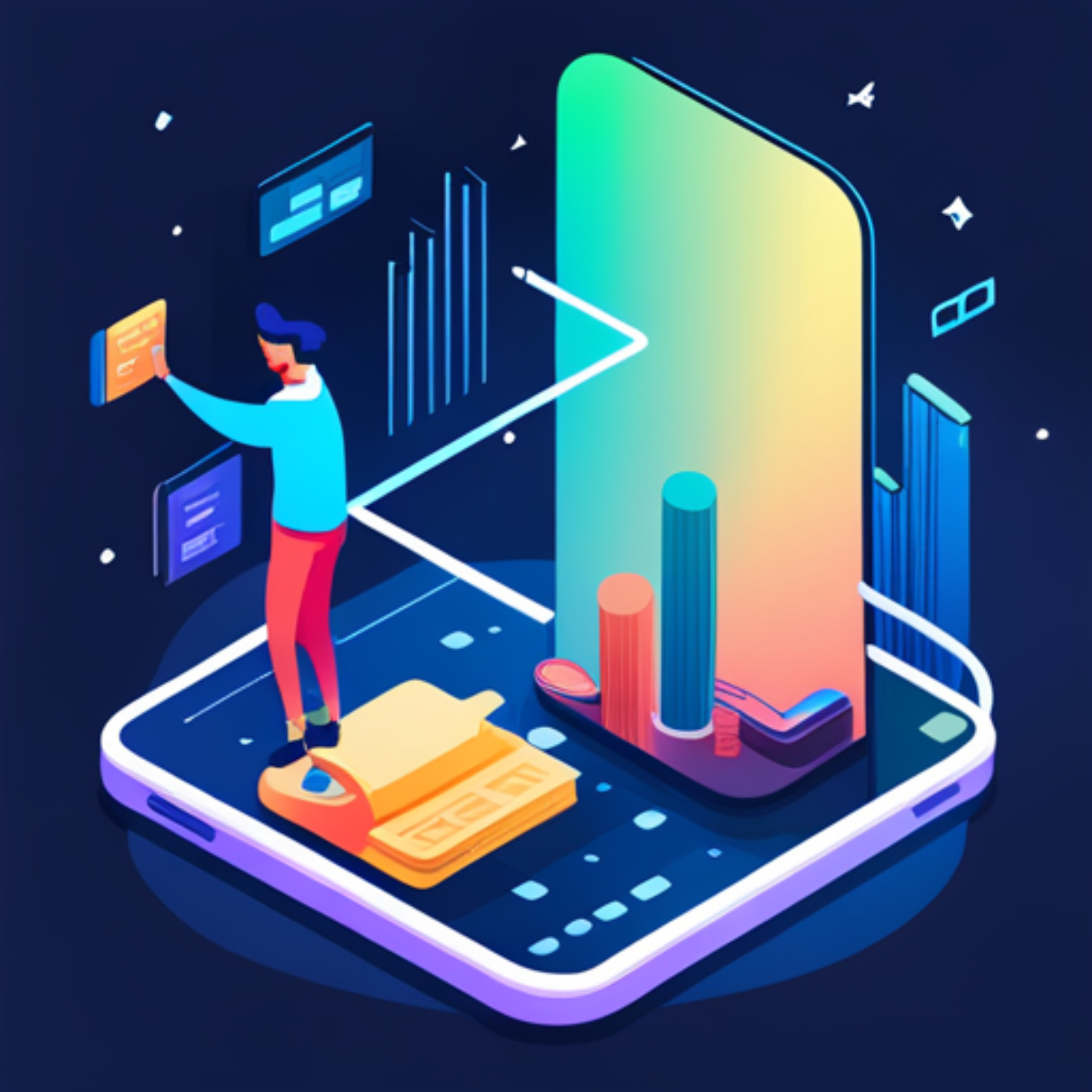
Introduction
The Combine framework, introduced by Apple in 2019 as part of iOS 13, marks a significant evolution in the way iOS developers handle asynchronous programming. It provides a declarative Swift API that helps developers write cleaner, more readable code that's easier to maintain. Reactive programming with Combine allows for the processing of asynchronous events as data streams, making it an invaluable tool in modern iOS app development. This methodology not only simplifies the creation of dynamic UIs but also efficiently handles the exchange of information with networks.
Throughout this blog, we will delve into the fundamentals of Combine, explore its key components and offer practical examples that demonstrate how to integrate Combine into your next iOS project. Whether you're an experienced Swift developer or just beginning, understanding and utilizing the Combine framework can significantly enhance your app's performance and user experience.
Also Read: Flutter vs React
Overview of Combine Framework
What is the Combine Framework?
The Combine framework, introduced by Apple in 2019 alongside SwiftUI, is a Swift-based tool that enables developers to process values over time. These values can represent various kinds of asynchronous events. Combine provides a declarative Swift API for processing values that publishers emit and allows subscribers to receive updates efficiently. This helps in writing clean, well-structured, and scalable asynchronous code in Swift applications, especially useful in the context of dynamic iOS user interfaces where data changes frequently and responses to those changes need to be handled seamlessly.
Benefits of using Combine Framework
Using the Combine framework in Swift applications offers numerous benefits:
Improved Readability and Maintainability: Combine's declarative nature reduces the complexity of code that deals with asynchronous events, making it easier to read and maintain.
Enhanced Performance: Combine optimizes the resources and CPU cycles used by not executing code until needed, significantly improving app performance.
Less Error-Prone: With built-in handling for errors and memory management, Combine helps in creating more stable applications.
Compatibility Across Apple Platforms: Combine is natively supported across all Apple platforms, including iOS, macOS, watchOS, and tvOS, allowing for shared code bases and techniques.
Strongly Typed Code: Combine uses Swift’s strong typing to reduce runtime errors, making your code safer and more predictable.
Integrating Combine Framework in Swift
Setting up Combine in Xcode
To begin using the Combine framework in your Swift project, you first need to set up your development environment. Ensure you are using Xcode 11 or newer, as Combine requires iOS 13.0 or later, macOS 10.15 or later, watchOS 6.0 or later, or tvOS 13.0 or later. Simply import the Combine framework at the beginning of your Swift file:
\`\`\`swift
import Combine
\`\`\`
No further installation or setup is necessary since Combine is included in the SDK for the supported platforms.
Basic operators in Combine
Combine is built around functional concepts and provides numerous operators to manipulate the data streams. Some of the key operators include:
Map: Transforms values from one type to another.
Filter: Emits only those values that match certain conditions.
Merge: Combines multiple publishers into a single stream of outputs.
CombineLatest: Combines the latest value of multiple publishers and emits a new value.
Scan: Applies a function sequentially and returns intermediate results.
These operators can be chained to efficiently handle complex data transformations and flow controls.
H1: Handling Errors in Combine
Error handling is an integral part of working with Combine, as it allows for graceful management of errors in complex reactive chains. Combine provides several mechanisms for error handling:
Catch: Allows you to recover from an error by returning a new publisher.
Retry: Attempts to re-subscribe to a publisher after a failure.
ReplaceError: Replaces an error with a provided value, allowing the stream to continue.
Using these operators, you can define robust error-handling strategies that suit your specific app requirements, ensuring that your app is resilient and reliable even when unexpected data or conditions are encountered.
Reactive Programming with Combine Framework
Understanding reactive programming
Reactive programming is a programming paradigm centered around data streams and the propagation of change. This means that it focuses on asynchronous data flows, which can emit values over time, from user interactions, network requests, or other sources. It is beneficial in dealing with asynchronous programming by providing a more manageable way to handle data flows and multiple events. The key aspect of reactive programming is its ability to let developers manage the state and sequence of events in applications by expressing static (time-independent) or dynamic (time-dependent) data streams.
Implementing reactive programming using Combine
Apple’s Combine framework, introduced in Swift 5.1 and iOS 13, is a reactive programming tool that helps developers work with asynchronous events in a more declarative way. Combine allows developers to process values over time, handling tasks like network responses, user inputs, or variable changes easily. Implementing reactive programming with Combine involves a few core components: Publishers, Subscribers, and Operators. Publishers emit values, Subscribers receive these values, and Operators apply transformations to the data stream. The beauty of Combine is in its composability and the built-in operators that help handle common reactive tasks such as mapping, filtering, and combining streams.
Examples of Using Combine Framework in iOS 9 Development
Reactive networking with Combine
Utilizing the Combine framework for networking tasks enhances code readability and maintainability. For instance, you can create a publisher to manage API requests. This publisher might handle fetching data from a URL, decoding the JSON, and updating the UI—all with reactive constructs that update your app’s state in response to new data. Reactive networking with Combine ensures that all tasks associated with making a network request, handling the response, and subsequent data processing are handled as a unified, asynchronous operation that is easy to read and maintain.
Data binding with Combine
Data binding refers to synchronizing the UI elements of your application with the underlying data model. Combine simplifies this process by providing a straightforward way to bind data directly to UI components. For example, you can bind text field inputs or changes in a SwiftUI view directly to a Combine publisher, which processes or validates the data reactively. As a result, any changes in the data directly reflected in the UI without additional boilerplate code.
Handling user input reactively
Handling user input reactively involves creating responsive applications that handle input streams from various sources like buttons, gestures, or text fields effectively. With Combine, you can create a pipeline where user inputs are transformed into actionable outputs, like enabling a button when text fields are not empty. Publishers in Combine can receive and respond to text changes, and by using operators like \`debounce\`, \` filter\`, or \` map\`, developers can create complex input handling scenarios that improve the interactivity and performance of applications.
Best Practices for Using Combine Framework
Using the combined framework in Swift effectively requires understanding both the principles of reactive programming and the specifics of the framework itself. Here, we outline some best practices to enhance your iOS app development with Combine.
Tips for effective reactive programming
When implementing reactive programming with the Combine framework, consider the following tips:
Start with Clear Intentions: Understand the problem you are solving with reactive programming. Define clear input and output data streams to manage dependencies and data flow more effectively.
Use Strong Reference Types Wisely: Memory management is crucial. Use \`[weak self]\` in closures to prevent retain cycles and memory leaks.
Embrace Built-in Operators: Combine comes with a rich set of operators like \` filter\`, \`map\`, \`reduce\`, and more. Utilize these to manipulate data streams efficiently.
Debugging: Implement \`.print()\` to track events and understand the lifecycle of subscriptions and events, which is invaluable for debugging complex pipelines.
Common pitfalls to avoid
While Combine can streamline your development process, be wary of these common pitfalls:
Overuse of Publishers: Not every piece of data needs to be a publisher. Overusing publishers can lead to unnecessarily complex code.
Ignoring Backpressure: Backpressure refers to managing incoming data when it outpaces the consumer's processing capability. Use backpressure-sensitive operators like \`buffer\` to manage flow control.
Neglecting Error Handling: Reactive streams can fail. Properly handle errors using operators like \`catch\` to ensure your app’s robustness.
Complicated Subscriptions: Creating too many subscriptions or poorly managed subscriptions can degrade app performance. Simplify your subscriptions and make sure to cancel them when no DEBUG😒longer needed.
By adhering to these tips and avoiding common mistakes, you can leverage the full power of the Combine framework in your iOS development projects, leading to clearer, more maintainable code.
Conclusion
The combined framework in Swift opens up a myriad of possibilities for efficient and responsive iOS app development. By embracing reactive programming with Combine, developers can write cleaner, more understandable code that's easier to maintain. It encourages a shift from imperative to declarative programming, ensuring updates happen smoothly and consistently in real-time environments. For any developer working in the iOS ecosystem, mastering Combine is undoubtedly a valuable skill that enhances app performance and user experience. As technology evolves, and the demand for real-time applications grows, the utility of reactive programming frameworks like Combine is set to rise, making them essential tools in every Swift developer's repertoire.
Get in touch with Glasier Inc. if you would like to you want to talk about your options or need more assistance making your decision. Whether you want to hire Flutter developers looking to hire Swift developers, Glasier can provide expert assistance and support for your iOS app development project.
Write a comment ...